tiny_hello.py
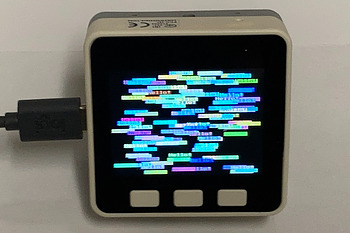
Test text_font_converter on small displays.
Writes “Hello!” in a tiny font in random colors at random locations on the Display.
Note
This example requires the following modules:
|
|
|
1"""
2tiny_hello.py
3=============
4
5.. figure:: ../_static/tiny_hello.jpg
6 :align: center
7
8 Test text_font_converter on small displays.
9
10Writes "Hello!" in a tiny font in random colors at random locations on the Display.
11
12.. note:: This example requires the following modules:
13
14 .. hlist::
15 :columns: 3
16
17 - `st7789py`
18 - `tft_config`
19 - `vga1_8x8`
20
21"""
22
23import random
24import time
25
26import st7789py as st7789
27import tft_config
28import vga1_8x8 as font
29
30tft = tft_config.config(tft_config.WIDE)
31
32
33def center(text, fg=st7789.WHITE, bg=st7789.BLACK):
34 """
35 Centers the given text on the display.
36 """
37 length = len(text)
38 tft.text(
39 font,
40 text,
41 tft.width // 2 - length // 2 * font.WIDTH,
42 tft.height // 2 - font.HEIGHT,
43 fg,
44 bg,
45 )
46
47
48def main():
49 """
50 The big show!
51 """
52 for color in [st7789.RED, st7789.GREEN, st7789.BLUE]:
53 tft.fill(color)
54 tft.rect(0, 0, tft.width, tft.height, st7789.WHITE)
55 center("Hello!", st7789.WHITE, color)
56 time.sleep(1)
57
58 while True:
59 for rotation in range(4):
60 tft.rotation(rotation)
61 tft.fill(0)
62 col_max = tft.width - font.WIDTH * 6
63 row_max = tft.height - font.HEIGHT
64
65 for _ in range(128):
66 tft.text(
67 font,
68 "Hello!",
69 random.randint(0, col_max),
70 random.randint(0, row_max),
71 st7789.color565(
72 random.getrandbits(8),
73 random.getrandbits(8),
74 random.getrandbits(8),
75 ),
76 st7789.color565(
77 random.getrandbits(8),
78 random.getrandbits(8),
79 random.getrandbits(8),
80 ),
81 )
82
83
84main()