noto_fonts.py
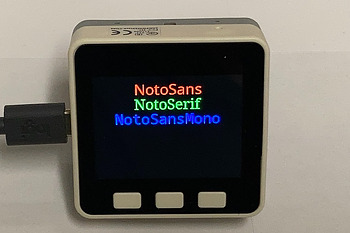
Test for TrueType write_font_converter.
Writes the names of three Noto fonts centered on the display using the font. The fonts were converted from True Type fonts using the write_font_converter.py utility.
Note
This example requires the following modules:
|
|
|
1"""
2noto_fonts.py
3=============
4
5.. figure:: ../_static/noto_fonts.jpg
6 :align: center
7
8 Test for TrueType write_font_converter.
9
10Writes the names of three Noto fonts centered on the display using the font.
11The fonts were converted from True Type fonts using the
12:ref:`write_font_converter.py<write_font_converter>` utility.
13
14.. note:: This example requires the following modules:
15
16 .. hlist::
17 :columns: 3
18
19 - `st7789py`
20 - `tft_config`
21 - `NotoSans_32`
22 - `NotoSerif_32`
23 - `NotoSansMono_32`
24
25"""
26
27import tft_config
28import st7789py as st7789
29import NotoSans_32 as noto_sans
30import NotoSerif_32 as noto_serif
31import NotoSansMono_32 as noto_mono
32
33
34def main():
35 """ main """
36
37 def center(font, string, row, color=st7789.WHITE):
38 """
39 Centers the given string horizontally on the screen at the specified row.
40
41 Args:
42 font: The font to use for rendering the string.
43 string: The string to be centered.
44 row: The row where the string will be displayed.
45 color: The color of the string (default: st7789.WHITE).
46
47 Returns:
48 None
49 """
50
51 screen = tft.width # get screen width
52 width = tft.write_width(font, string) # get the width of the string
53 col = tft.width // 2 - width // 2 if width and width < screen else 0
54 tft.write(font, string, col, row, color) # and write the string
55
56 # initialize the display
57 tft = tft_config.config(tft_config.WIDE)
58 row = 16
59
60 # center the name of the first font, using the font
61 center(noto_sans, "NotoSans", row, st7789.RED)
62 row += noto_sans.HEIGHT
63
64 # center the name of the second font, using the font
65 center(noto_serif, "NotoSerif", row, st7789.GREEN)
66 row += noto_serif.HEIGHT
67
68 # center the name of the third font, using the font
69 center(noto_mono, "NotoSansMono", row, st7789.BLUE)
70 row += noto_mono.HEIGHT
71
72
73main()