scroll.py
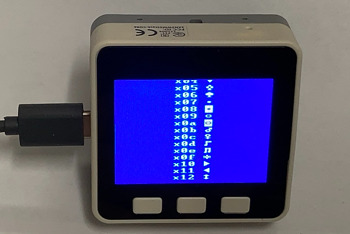
Test for hardware scrolling.
Smoothly scrolls all font characters up the screen. Only works with fonts with heights that are even multiples of the screen height, (i.e. 8 or 16 pixels high)
Note
This example requires the following modules:
|
|
|
1"""
2scroll.py
3=========
4
5.. figure:: ../_static/scroll.jpg
6 :align: center
7
8 Test for hardware scrolling.
9
10Smoothly scrolls all font characters up the screen.
11Only works with fonts with heights that are even multiples of the screen height,
12(i.e. 8 or 16 pixels high)
13
14.. note:: This example requires the following modules:
15
16 .. hlist::
17 :columns: 3
18
19 - `st7789py`
20 - `tft_config`
21 - `vga2_bold_16x16`
22
23"""
24
25import utime
26import random
27import st7789py as st7789
28import tft_config
29import vga2_bold_16x16 as font
30
31
32def main():
33 """ main """
34 tft = tft_config.config(tft_config.SCROLL)
35 last_line = tft.height - font.HEIGHT
36 tfa = tft_config.TFA # top free area when scrolling
37 bfa = tft_config.BFA # bottom free area when scrolling
38 tft.vscrdef(tfa, 240, bfa)
39
40 tft.fill(st7789.BLUE)
41 scroll = 0
42 character = 0
43 col = tft.width // 2 - 5 * font.WIDTH // 2
44
45 while True:
46 tft.fill_rect(0, scroll, tft.width, 1, st7789.BLUE)
47
48 if scroll % font.HEIGHT == 0:
49 tft.text(
50 font,
51 f'x{character:02x} {chr(character)}',
52 col,
53 (scroll + last_line) % tft.height,
54 st7789.WHITE,
55 st7789.BLUE)
56
57 character = character + 1 if character < 256 else 0
58
59 tft.vscsad(scroll + tfa)
60 scroll += 1
61
62 if scroll == tft.height:
63 scroll = 0
64
65 utime.sleep(0.01)
66
67
68main()