rotations.py
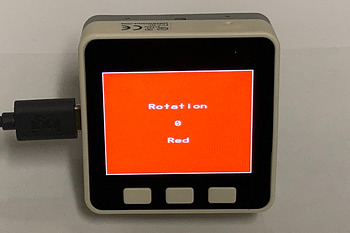
Test for rotations and colors.
Rotates the display 0, 90, 180, and 270 degrees and displays the rotation number and the color of the display background.
Note
This example requires the following modules:
|
|
|
1"""
2rotations.py
3============
4
5.. figure:: ../_static/rotations.jpg
6 :align: center
7
8 Test for rotations and colors.
9
10Rotates the display 0, 90, 180, and 270 degrees and displays the rotation
11number and the color of the display background.
12
13.. note:: This example requires the following modules:
14
15 .. hlist::
16 :columns: 3
17
18 - `st7789py`
19 - `tft_config`
20 - vga1_16x16
21
22"""
23
24import time
25import tft_config
26import st7789py as st7789
27import vga1_16x16 as font
28
29
30def center_on(display, using_font, text, y, fg, bg):
31 """
32 Center the text on the display
33 """
34 x = (display.width - len(text) * font.WIDTH) // 2
35 display.text(using_font, text, x, y, fg, bg)
36
37def clear_screen(display, color):
38 """
39 Clear the screen by drawing rectangles starting from the center of the display
40 and working towards the edges.
41
42 Args:
43 display: The display object to clear.
44 """
45 width = display.width
46 height = display.height
47 x_center = width // 2
48 y_center = height // 2
49
50 for i in range(min(x_center, y_center)):
51 x = x_center - i
52 y = y_center - i
53 rect_width = 2 * i + 1
54 rect_height = 2 * i + 1
55 display.rect(x, y, rect_width, rect_height, color)
56
57def main():
58 """
59 The big show!
60 """
61 # enable display and clear screen
62
63 tft = tft_config.config(tft_config.WIDE)
64
65 colors = (
66 ("Red", st7789.RED, st7789.WHITE),
67 ("Green", st7789.GREEN, st7789.BLACK),
68 ("Blue", st7789.BLUE, st7789.WHITE),
69 ("Black", st7789.BLACK, st7789.WHITE),
70 ("White", st7789.WHITE, st7789.BLACK),
71 ("Yellow", st7789.YELLOW, st7789.BLACK),
72 ("Cyan", st7789.CYAN, st7789.BLACK),
73 ("Magenta", st7789.MAGENTA, st7789.BLACK),
74 )
75
76 color_idx = 0
77 while True:
78 for rotation in range(4):
79 tft.rotation(rotation)
80 height = tft.height
81 width = tft.width
82 fg = colors[color_idx][2]
83 bg = colors[color_idx][1]
84
85 tft.fill(bg)
86
87 tft.rect(0, 0, width, height, st7789.WHITE)
88 center_on(tft, font, "Rotation", height // 3 - font.HEIGHT // 2, fg, bg)
89 center_on(tft, font, str(rotation), height // 2 - font.HEIGHT // 2, fg, bg)
90 center_on(
91 tft,
92 font,
93 colors[color_idx][0],
94 height // 3 * 2 - font.HEIGHT // 2,
95 fg,
96 bg,
97 )
98 color_idx = (color_idx + 1) % len(colors)
99 time.sleep(2)
100
101main()