color_test.py
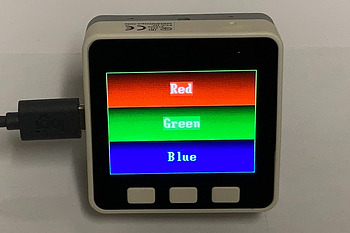
Test color with gradients.
Draws Red, Green and Blues gradients on the display and writes the color name centered in the gradient. Then repeatedly draws a borders around the display in the same colors.
Note
This example requires the following modules:
|
|
|
1"""
2color_test.py
3=============
4
5.. figure:: ../_static/color_test.jpg
6 :align: center
7
8 Test color with gradients.
9
10Draws Red, Green and Blues gradients on the display and writes the color name centered in the
11gradient. Then repeatedly draws a borders around the display in the same colors.
12
13.. note:: This example requires the following modules:
14
15 .. hlist::
16 :columns: 3
17
18 - `st7789py`
19 - `tft_config`
20 - `vga2_bold_16x32`
21
22"""
23
24import random
25from machine import Pin, SoftSPI
26from time import sleep
27import st7789py as st7789
28import tft_config
29import vga2_bold_16x32 as font
30
31
32def interpolate(value1, value2, position, total_range):
33 """
34 Perform linear interpolation between two values based on a position within a range.
35
36 Args:
37 value1 (float): Starting value.
38 value2 (float): Ending value.
39 position (float): Current position within the range.
40 total_range (float): Total range of positions.
41
42 Returns:
43 float: Interpolated value.
44 """
45 return value1 + (value2 - value1) * position / total_range
46
47
48def main():
49 tft = tft_config.config(tft_config.WIDE)
50
51 names = ["Red", "Green", "Blue"]
52
53 color_values = (255, 255, 255)
54 height_division = tft.height // len(color_values)
55 for i, color_value in enumerate(color_values):
56 start_row = i * height_division
57 end_row = (i + 1) * height_division
58 for row in range(start_row, end_row):
59 rgb_color = [0 if idx != i else int(interpolate(0, color_value, row - start_row, height_division)) for idx in range(3)]
60 color = st7789.color565(rgb_color)
61 tft.hline(0, row, tft.width, color)
62
63 name = names[i]
64 text_x = (tft.width - font.WIDTH * len(name)) // 2
65 text_y = start_row + (end_row - start_row - font.HEIGHT) // 2
66 tft.text(font, name, text_x, text_y, st7789.WHITE, color)
67
68 while True:
69 for color in [st7789.RED, st7789.GREEN, st7789.BLUE]:
70 for x in range(tft.width):
71 tft.pixel(x, 0, color)
72 tft.pixel(x, tft.height - 1, color)
73
74 for y in range(tft.height):
75 tft.pixel(0 , y, color)
76 tft.pixel(tft.width - 1, y, color)
77
78 sleep(1)
79main()