feathers.py
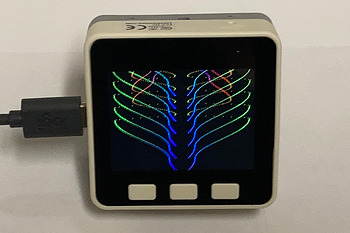
Test hardware scrolling.
Smoothly scrolls mirrored rainbow colored random curves across the display.
Note
This example requires the following modules:
|
|
1"""
2feathers.py
3===========
4
5.. figure:: ../_static/feathers.jpg
6 :align: center
7
8 Test hardware scrolling.
9
10Smoothly scrolls mirrored rainbow colored random curves across the display.
11
12.. note:: This example requires the following modules:
13
14 .. hlist::
15 :columns: 3
16
17 - `st7789py`
18 - `tft_config`
19
20"""
21
22import random
23import math
24import time
25import st7789py as st7789
26import tft_config
27
28
29def between(left, right, along):
30 """returns a point along the curve from left to right"""
31 dist = (1 - math.cos(along * math.pi)) / 2
32 return left * (1 - dist) + right * dist
33
34
35def color_wheel(position):
36 """returns a 565 color from the given position of the color wheel"""
37 position = (255 - position) % 255
38
39 if position < 85:
40 return st7789.color565(255 - position * 3, 0, position * 3)
41
42 if position < 170:
43 position -= 85
44 return st7789.color565(0, position * 3, 255 - position * 3)
45
46 position -= 170
47 return st7789.color565(position * 3, 255 - position * 3, 0)
48
49
50def main():
51 '''
52 The big show!
53 '''
54 #enable display and clear screen
55
56 tft = tft_config.config(tft_config.FEATHERS)
57
58 height = tft.height # height of display in pixels
59 width = tft.width # width if display in pixels
60
61 tfa = tft_config.TFA # top free area when scrolling
62 bfa = tft_config.BFA # bottom free area when scrolling
63
64 scroll = 0 # scroll position
65 wheel = 0 # color wheel position
66
67 tft.vscrdef(tfa, width, bfa) # set scroll area
68 tft.vscsad(scroll + tfa) # set scroll position
69 tft.fill(st7789.BLACK) # clear screen
70
71 half = (height >> 1) - 1 # half the height of the dislay
72 interval = 0 # steps between new points
73 increment = 0 # increment per step
74 counter = 1 # step counter, overflow to start
75 current_y = 0 # current_y value (right point)
76 last_y = 0 # last_y value (left point)
77
78 # segment offsets
79 x_offsets = [x * (width // 8) -1 for x in range(2,9)]
80
81 while True:
82 # when the counter exceeds the interval, save current_y to last_y,
83 # choose a new random value for current_y between 0 and 1/2 the
84 # height of the display, choose a new random interval then reset
85 # the counter to 0
86
87 if counter > interval:
88 last_y = current_y
89 current_y = random.randint(0, half)
90 counter = 0
91 interval = random.randint(10, 100)
92 increment = 1/interval # increment per step
93
94 # clear the first column of the display and scroll it
95 tft.vline(scroll, 0, height, st7789.BLACK)
96 tft.vscsad(scroll + tfa)
97
98 # get the next point between last_y and current_y
99 tween = int(between(last_y, current_y, counter * increment))
100
101 # draw mirrored pixels across the display at the offsets using the color_wheel effect
102 for i, x_offset in enumerate(x_offsets):
103 tft.pixel((scroll + x_offset) % width, half + tween, color_wheel(wheel+(i<<2)))
104 tft.pixel((scroll + x_offset) % width, half - tween, color_wheel(wheel+(i<<2)))
105
106 # increment scroll, counter, and wheel
107 scroll = (scroll + 1) % width
108 wheel = (wheel + 1) % 256
109 counter += 1
110
111
112main()