boxlines.py
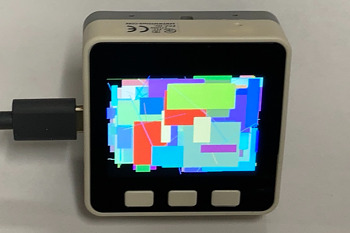
Test for lines and rectangles.
Draws lines and rectangles in random colors at random locations on the display.
Note
This example requires the following modules:
|
|
1"""
2boxlines.py
3===========
4
5.. figure:: ../_static/boxlines.jpg
6 :align: center
7
8 Test for lines and rectangles.
9
10Draws lines and rectangles in random colors at random locations on the display.
11
12.. note:: This example requires the following modules:
13
14 .. hlist::
15 :columns: 3
16
17 - `st7789py`
18 - `tft_config`
19
20"""
21
22import random
23import tft_config
24import st7789py as st7789
25
26
27def main():
28 """ main """
29 tft = tft_config.config(tft_config.WIDE)
30
31 while True:
32 color = st7789.color565(
33 random.getrandbits(8), random.getrandbits(8), random.getrandbits(8)
34 )
35
36 tft.line(
37 random.randint(0, tft.width),
38 random.randint(0, tft.height),
39 random.randint(0, tft.width),
40 random.randint(0, tft.height),
41 color,
42 )
43
44 width = random.randint(0, tft.width // 2)
45 height = random.randint(0, tft.height // 2)
46 col = random.randint(0, tft.width - width)
47 row = random.randint(0, tft.height - height)
48 tft.fill_rect(
49 col,
50 row,
51 width,
52 height,
53 st7789.color565(
54 random.getrandbits(8), random.getrandbits(8), random.getrandbits(8)
55 ),
56 )
57
58
59main()