alien.py
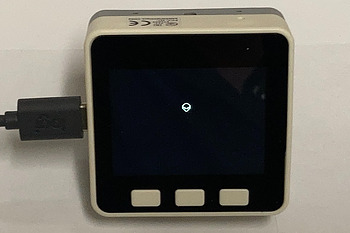
Bounce a bitmap of an alien around the display.
The alien_bitmap module was created using the image_converter.py utility.
#!/bin/sh
../../utils/image_converter.py alien.png 1 >alien_bitmap.py
# mpy-cross alien_bitmap.py
# mpremote cp alien_bitmap.mpy :
# mpremote run alien.py
Note
This example requires the following modules:
|
|
|
The alien.png PNG file is from the Erik Flowers Weather Icons available from https://github.com/erikflowers/weather-icons and is licensed under SIL OFL 1.1 (http://scripts.sil.org/OFL).
1"""
2alien.py
3=========
4
5.. figure:: ../_static/alien.jpg
6 :align: center
7
8 Bounce a bitmap of an alien around the display.
9
10The alien_bitmap module was created using the :ref:`image_converter.py<image_converter>` utility.
11
12.. literalinclude:: ../../../examples/alien/make_alien_bitmap.sh
13
14.. note:: This example requires the following modules:
15
16 .. hlist::
17 :columns: 3
18
19 - `st7789py`
20 - `tft_config`
21 - `alien_bitmap`
22
23The alien.png PNG file is from the Erik Flowers Weather Icons available from
24https://github.com/erikflowers/weather-icons and is licensed under SIL OFL 1.1
25(http://scripts.sil.org/OFL).
26
27"""
28
29import time
30import st7789py as st7789
31import tft_config
32import alien_bitmap as alien
33
34SPEED_X = 3
35SPEED_Y = 2
36TICKS = 100
37
38
39def main():
40 """
41 Runs the main loop for the bounce animation.
42 """
43
44 tft = tft_config.config(tft_config.WIDE)
45 width, height = tft.width, tft.height
46 col, row = width // 2 - alien.WIDTH // 2, height // 2 - alien.HEIGHT // 2
47 xd, yd = SPEED_X, SPEED_Y
48 last_col, old_row = col, row
49
50 while True:
51 last = time.ticks_ms()
52 tft.fill_rect(last_col, old_row, alien.WIDTH, alien.HEIGHT, 0)
53 tft.bitmap(alien, col, row)
54 last_col, old_row = col, row
55 col, row = col + xd, row + yd
56 xd = -xd if col <= 0 or col >= width - alien.WIDTH else xd
57 yd = -yd if row <= 0 or row >= height - alien.HEIGHT else yd
58
59 if time.ticks_ms() - last < TICKS:
60 time.sleep_ms(TICKS - (time.ticks_ms() - last))
61
62
63main()