toasters.py
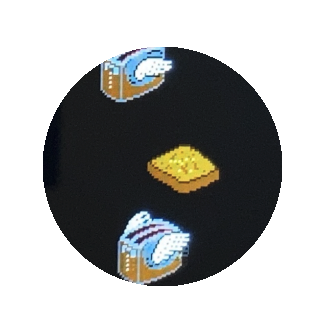
An example using bitmap to draw sprites on the display.
- Spritesheet from CircuitPython_Flying_Toasters
https://learn.adafruit.com/circuitpython-sprite-animation-pendant-mario-clouds-flying-toasters
1"""
2toasters.py
3===========
4
5.. figure:: /_static/toasters.png
6 :align: center
7
8 An example using bitmap to draw sprites on the display.
9
10Spritesheet from CircuitPython_Flying_Toasters
11 https://learn.adafruit.com/circuitpython-sprite-animation-pendant-mario-clouds-flying-toasters
12"""
13
14import time
15import random
16import gc9a01
17import tft_config
18
19import t1, t2, t3, t4, t5
20
21TOASTERS = [t1, t2, t3, t4]
22TOAST = [t5]
23
24
25class Toast:
26 """
27 toast class to keep track of a sprites locaton and step
28 """
29
30 def __init__(self, sprites, x, y, width, height):
31 self.sprites = sprites
32 self.steps = len(sprites)
33 self.x = x
34 self.y = y
35 self.step = random.randint(0, self.steps - 1)
36 self.speed = random.randint(2, 5)
37 self.width = width
38 self.height = height
39
40 def move(self):
41 """
42 Moves an object horizontally on the screen. If the object reaches the left
43 edge of the screen, it resets its position and speed.
44 """
45 if self.x <= 0:
46 self.speed = random.randint(2, 5)
47 self.x = self.width - 64
48
49 self.step += 1
50 self.step %= self.steps
51 self.x -= self.speed
52
53
54def main():
55 """
56 Draw and move sprite
57 """
58 tft = tft_config.config(tft_config.TALL)
59
60 # enable display and clear screen
61 tft.init()
62 tft.fill(gc9a01.BLACK)
63
64 # cache width and height
65 width = tft.width()
66 height = tft.height()
67
68 # create toast spites in random positions
69 sprites = [
70 Toast(TOASTERS, width - 64, 0, width, height),
71 Toast(TOAST, width - 64 * 2, 80, width, height),
72 Toast(TOASTERS, width - 64 * 4, 160, width, height),
73 ]
74
75 # move and draw sprites
76 while True:
77 for man in sprites:
78 bitmap = man.sprites[man.step]
79 tft.fill_rect(
80 man.x + bitmap.WIDTH - man.speed,
81 man.y,
82 man.speed,
83 bitmap.HEIGHT,
84 gc9a01.BLACK,
85 )
86
87 man.move()
88
89 if man.x > 0:
90 tft.bitmap(bitmap, man.x, man.y)
91 else:
92 tft.fill_rect(0, man.y, bitmap.WIDTH, bitmap.HEIGHT, gc9a01.BLACK)
93
94 time.sleep(0.05)
95
96
97main()