hershey.py
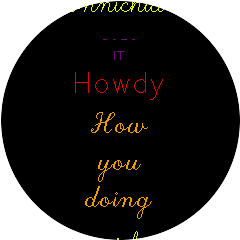
Hershey vector font demo
Demo program that draws greetings on display cycling thru hershey fonts and colors.
1"""
2hershey.py
3==========
4.. figure:: /_static/hershey.png
5 :align: center
6
7 Hershey vector font demo
8
9Demo program that draws greetings on display cycling thru hershey fonts and colors.
10"""
11
12import random
13import utime
14import gc9a01
15import tft_config
16
17tft = tft_config.config(tft_config.WIDE, options=gc9a01.WRAP_V)
18
19# Load several frozen fonts from flash
20
21import greeks
22import italicc
23import italiccs
24import meteo
25import romanc
26import romancs
27import romand
28import romanp
29import romans
30import scriptc
31import scripts
32
33
34def cycle(p):
35 """return the next item in a list"""
36 try:
37 len(p)
38 except TypeError:
39 cache = []
40 for i in p:
41 yield i
42 cache.append(i)
43
44 p = cache
45 while p:
46 yield from p
47
48
49# Create a cycle of colors
50COLORS = cycle([0xe000, 0xece0, 0xe7e0, 0x5e0, 0x00d3, 0x7030])
51
52# List Hershey fonts
53FONT = [greeks, italicc, italiccs, meteo, romanc, romancs, romand, romanp, romans, scriptc, scripts]
54
55# Create a cycle of tuples consisting of a FONT[] and the HEIGHT of the next FONT[] in the cycle
56FONTS = cycle([
57 (font, FONT[(i + 1) % len(FONT)].HEIGHT)
58 for i, font in enumerate(FONT)])
59
60# Greetings
61GREETING = [
62 "Ahoy", "Bonjour", "Bonsoir", "Buenos Dias", "Buenas tardes", "Buenas Noches", "Ciao", "Dude",
63 "Good Morning", "Good Day", "Good Evening", "Hello", "Hey", "Hi", "Hiya", "Hola", "How Are You",
64 "How Goes It", "Howdy", "How you doing", "Konnichiwa","Salut", "Shalom", "Welcome", "What's Up",
65 'Yo!']
66
67# Create a cycle of tuples consisting of a list of words from a GREETING, the number of spaces+1
68# in the that GREETING, and the number of spaces+1 in the next GREETING of the cycle
69GREETINGS = cycle([
70 (greeting.split(), greeting.count(' ')+1, GREETING[(i + 1) % len(GREETING)].count(' ')+1)
71 for i, greeting in enumerate(GREETING)])
72
73
74def main():
75 """Scroll greetings on the display cycling thru Hershey fonts and colors"""
76
77 tft.init()
78 tft.fill(gc9a01.BLACK)
79
80 height = tft.height()
81 width = tft.width()
82
83 # Set up scrolling area
84 tfa = tft_config.TFA
85 bfa = tft_config.BFA
86 tft.vscrdef(tfa, height, bfa)
87
88 scroll = 0
89 to_scroll = 0
90
91 while True:
92
93 # if we have scrolled high enough for the next greeting
94 if to_scroll == 0:
95 font = next(FONTS) # get the next font
96 greeting = next(GREETINGS) # get the next greeting
97 color = next(COLORS) # get the next color
98 lines = greeting[2] # number of lines in the greeting
99 to_scroll = lines * font[1] + 8 # number of rows to scroll
100
101 # draw each line of the greeting
102 for i, word in enumerate(greeting[0][::-1]):
103 word_len = tft.draw_len(font[0], word) # width in pixels
104 col = 0 if word_len > width else (width//2 - word_len//2) # column to center
105 row = (scroll + height - ((i + 1) * font[0].HEIGHT) % height) # row to draw
106 tft.draw(font[0], word, col, row, color) # draw the word
107
108 tft.fill_rect(0, scroll, width, 1, gc9a01.BLACK) # clear the top line
109 tft.vscsad(scroll+tfa) # scroll the display
110 scroll = (scroll+1) % height # update the scroll position
111 to_scroll -= 1 # update rows left to scroll
112 utime.sleep(0.02) # stop and smell the roses
113
114
115main()