hello_world.py
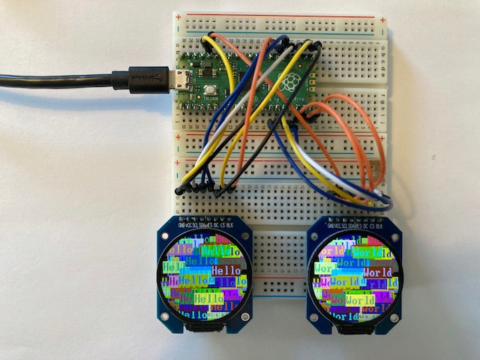
Dual display Hello World example.
Writes “Hello World” in random colors at random locations split across a pair of GC9A01 displays connected to a Raspberry Pi Pico.
1"""
2hello_world.py
3==============
4
5.. figure:: /_static/hello_world.png
6 :align: center
7
8 Dual display Hello World example.
9
10Writes "Hello World" in random colors at random locations split across a pair of GC9A01 displays
11connected to a Raspberry Pi Pico.
12
13"""
14
15from machine import Pin, SPI
16import random
17import gc9a01
18import tft_config0
19import tft_config1
20
21import vga1_bold_16x32 as font
22
23
24def main():
25 """
26 Initializes tft0 and tft1 displays and displays "Hello" and
27 "World" at random locations on both displays.
28 """
29 tft0 = tft_config0.config(tft_config0.TALL)
30 tft1 = tft_config1.config(tft_config1.TALL)
31
32 tft0.init()
33 tft1.init()
34
35 while True:
36 for rotation in range(4):
37 tft0.rotation(rotation)
38 tft0.fill(0)
39
40 tft1.rotation(rotation)
41 tft1.fill(0)
42
43 col_max = tft0.width() - font.WIDTH * 5
44 row_max = tft0.height() - font.HEIGHT
45
46 for _ in range(128):
47 col = random.randint(0, col_max)
48 row = random.randint(0, row_max)
49
50 fg = gc9a01.color565(
51 random.getrandbits(8), random.getrandbits(8), random.getrandbits(8)
52 )
53
54 bg = gc9a01.color565(
55 random.getrandbits(8), random.getrandbits(8), random.getrandbits(8)
56 )
57
58 tft0.text(font, "Hello", col, row, fg, bg)
59 tft1.text(font, "World", col, row, fg, bg)
60
61
62main()