lines.py
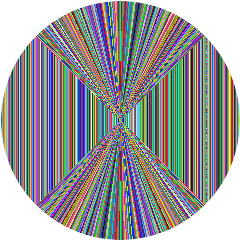
Benchmarks the speed of drawing horizontal and vertical lines on the display.
1"""
2lines.py
3========
4.. figure:: /_static/lines.png
5 :align: center
6
7Benchmarks the speed of drawing horizontal and vertical lines on the display.
8"""
9
10import random
11import time
12import gc9a01
13import tft_config
14
15tft = tft_config.config(tft_config.TALL)
16WIDTH = tft.width()
17HEIGHT = tft.height()
18
19
20def time_function(func, *args):
21 """time a function"""
22 start = time.ticks_ms()
23 func(*args)
24 return time.ticks_ms() - start
25
26
27def horizontal(line_count):
28 """draw line_count horizontal lines on random y positions"""
29 for _ in range(line_count):
30 y = random.randint(0, HEIGHT)
31 tft.line(
32 0,
33 y,
34 WIDTH,
35 y,
36 gc9a01.color565(
37 random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)
38 ),
39 )
40
41
42def vertical(line_count):
43 """draw line_count vertical lines on random x positions"""
44 for _ in range(line_count):
45 x = random.randint(0, WIDTH)
46 tft.line(
47 x,
48 0,
49 x,
50 HEIGHT,
51 gc9a01.color565(
52 random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)
53 ),
54 )
55
56
57def diagonal(line_count):
58 """draw line_count diagnal lines on random y positions"""
59 x = 0
60 for _ in range(line_count):
61 x += 1
62 x %= WIDTH
63 tft.line(
64 x,
65 0,
66 WIDTH - x,
67 HEIGHT,
68 gc9a01.color565(
69 random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)
70 ),
71 )
72
73
74def main():
75 tft.init()
76 tft.fill(0)
77 print("")
78 print("horizonal: ", time_function(horizontal, 1000))
79 print("vertical: ", time_function(vertical, 1000))
80 print("diagonal: ", time_function(diagonal, 1000))
81
82
83main()