proverbs.py
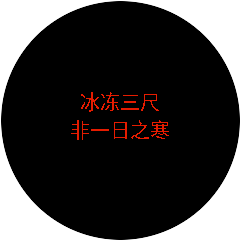
Test for TrueType write_font_converter.
Displays what I hope are chinese proverbs in simplified chinese to test UTF-8 font support. The fonts were converted from True Type fonts using the write_font_converter.py utility.
#!/bin/sh
SIZE=20
../../utils/write_font_converter.py \
-s "万事起头难。熟能生巧。冰冻三尺,非一日之寒。三个臭皮匠,胜过诸葛亮。今日事,今日毕。师父领进门,修行在个人。一口吃不成胖子。欲速则不达。百闻不如一见。不入虎穴,焉得虎子。" \
NotoSansSC-Regular.otf ${SIZE} >proverbs_${SIZE}.py
mpy-cross proverbs_${SIZE}.py
mpremote cp proverbs_${SIZE}.mpy :
Note
This example requires the following modules:
|
|
|
1"""
2proverbs.py
3===========
4
5.. figure:: /_static/proverbs.png
6 :align: center
7
8 Test for TrueType write_font_converter.
9
10Displays what I hope are chinese proverbs in simplified chinese to test UTF-8 font support.
11The fonts were converted from True Type fonts using the
12:ref:`write_font_converter.py<write_font_converter>` utility.
13
14.. literalinclude:: ../../examples/proverbs/make_proverbs_fonts.sh
15
16
17.. note:: This example requires the following modules:
18
19 .. hlist::
20 :columns: 3
21
22 - `gc9a01`
23 - `tft_config`
24 - `proverbs_20`
25
26"""
27
28import utime
29import gc9a01
30import tft_config
31import proverbs_20 as font
32
33
34def color_wheel(WheelPos):
35 """returns a 565 color from the given position of the color wheel"""
36 WheelPos = (255 - WheelPos) % 255
37
38 if WheelPos < 85:
39 return gc9a01.color565(255 - WheelPos * 3, 0, WheelPos * 3)
40
41 if WheelPos < 170:
42 WheelPos -= 85
43 return gc9a01.color565(0, WheelPos * 3, 255 - WheelPos * 3)
44
45 WheelPos -= 170
46 return gc9a01.color565(WheelPos * 3, 255 - WheelPos * 3, 0)
47
48
49def main():
50 proverbs = [
51 "万事起头难",
52 "熟能生巧",
53 "冰冻三尺 非一日之寒",
54 "三个臭皮匠 胜过诸葛亮",
55 "今日事 今日毕",
56 "师父领进门 修行在个人",
57 "一口吃不成胖子",
58 "欲速则不达",
59 "百闻不如一见",
60 "不入虎穴 焉得虎子",
61 ]
62
63 tft = tft_config.config(tft_config.WIDE)
64 tft.init()
65
66 line_height = font.HEIGHT + 8
67 half_height = tft.height() // 2
68 half_width = tft.width() // 2
69 wheel = 0
70
71 while True:
72 for proverb in proverbs:
73 proverb_lines = proverb.split(" ")
74 half_lines_height = len(proverb_lines) * line_height // 2
75 tft.fill(gc9a01.BLACK)
76
77 for count, proverb_line in enumerate(proverb_lines):
78 half_length = tft.write_len(font, proverb_line) // 2
79 tft.write(
80 font,
81 proverb_line,
82 half_width - half_length,
83 half_height - half_lines_height + count * line_height,
84 color_wheel(wheel),
85 )
86
87 wheel = (wheel + 5) % 256
88 # pause to slow down scrolling
89 utime.sleep(5)
90
91
92main()