arcs.py
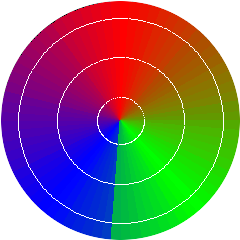
Arcs and Filled Arcs
Draw Arcs and Filled Arcs on the display.
1"""
2arcs.py
3=======
4
5.. figure:: /_static/arcs.png
6 :align: center
7
8 Arcs and Filled Arcs
9
10Draw Arcs and Filled Arcs on the display.
11"""
12
13import gc9a01
14import tft_config
15
16
17def color_wheel(step=1):
18 """Generator that yields a 565 color from the color wheel"""
19 position = 0
20 while True:
21 # Adjust the position within the range [0, 255)
22 adjusted_position = (255 - position) % 255
23
24 # Calculate the RGB values based on the adjusted position
25 if adjusted_position < 85:
26 r, g, b = 255 - adjusted_position * 3, 0, adjusted_position * 3
27 elif adjusted_position < 170:
28 adjusted_position -= 85
29 r, g, b = 0, adjusted_position * 3, 255 - adjusted_position * 3
30 else:
31 adjusted_position -= 170
32 r, g, b = adjusted_position * 3, 255 - adjusted_position * 3, 0
33
34 # Yield the next color in the wheel
35 yield gc9a01.color565(r, g, b)
36
37 # Move to the next position in the wheel
38 position = (position + step) % 255
39
40
41def main():
42 """
43 This function initializes the display and draws a series of colored arcs in a continuous loop.
44 """
45
46 # Initialize the TFT display and fill it with black color
47 tft = tft_config.config(tft_config.WIDE)
48 tft.init()
49 tft.fill(0)
50
51 # Get the width and height of the display
52 width, height = tft.width(), tft.height()
53
54 # Calculate the center of the display
55 center_x, center_y = width // 2, height // 2
56
57 # The radii of the arcs are equal to the half of the display's dimensions
58 radius_x, radius_y = center_x, center_y
59
60 # The step size for the angle of the arcs
61 step = 5
62
63 # Create a color wheel generator
64 wheel = color_wheel()
65
66 # Enter an infinite loop
67 while True:
68 # The start angle for the arcs
69 start_angle = 0
70
71 # Draw a series of arcs with increasing start and end angles
72 for end_angle in range(0, 361, step):
73 # Draw a filled arc with a color from the color wheel
74 tft.fill_arc(
75 center_x,
76 center_y,
77 start_angle,
78 end_angle,
79 5,
80 radius_x,
81 radius_y,
82 next(wheel),
83 )
84
85 # Draw a series of white arcs with with increasing radii
86 for fraction in range(20, 100, 33):
87 # Calculate the radius based on the fraction of the display's height
88 radius = int(fraction * center_y / 100 + 0.5)
89
90 # Draw a white arc
91 tft.arc(
92 center_x,
93 center_y,
94 start_angle,
95 end_angle,
96 step,
97 radius,
98 radius,
99 1,
100 gc9a01.WHITE,
101 )
102
103 # The start angle for the next arc is the end angle of the current arc
104 start_angle = end_angle
105
106
107# Call the main function
108main()